WordPress插件开发教程手册 — 用户、角色和能力
WordPress 用户存储在 wp_user 数据表中。
什么是用户?
WordPress 中,每个用户至少有一个用户名、密码和电子邮件地址。一旦创建了用户账户,用户就可以的登录后台来访问 WordPress 的功能和数据。
角色和能力
每个用户都会被分配一个角色,每个角色都会有一些能力。我们可以自定义一组能力来创建一个新角色。还可以创建自定义能力,然后分配给现有角色或新角色。在 WordPress 中,开发人员可以利用用户角色来限制用户是否可以执行一组操作。
最小能力原则
WordPress 遵守最少能力原则,即只赋予用户执行操作所需要的必不可少的能力的做法。
操作 WordPress 用户
添加用户
如果需要添加用户,我们可以使用 wp_create_user() 或 wp_insert_user() 函数。
wp_create_user() 使用用户名,密码和电子邮件参数创建用户,而 wp_insert_user() 接受描述用户及其属性的数组或对象。
创建用户
wp_create_user(
string $username,
string $password,
string $email = ''
);
上面的示例可以让我们创建一个新用户。
有关该函数参数的完整说明,请参阅关于 wp_create_user() 的函数参考。
创建用户的示例
// check if the username is taken
$user_id = username_exists($user_name);
// check that the email address does not belong to a registered user
if (!$user_id && email_exists($user_email) === false) {
// create a random password
$random_password = wp_generate_password(
$length = 12,
$include_standard_special_chars = false
);
// create the user
$user_id = wp_create_user(
$user_name,
$random_password,
$user_email
);
}
插入用户
wp_insert_user(
array|object|WP_User $userdata
);
有关该函数参数的完整说明,请参阅 wp_insert_user() 函数的参考文档。
插入用户示例
下面的示例演示了插入用户,并添加用户资料的过程。
$username = $_POST['username'];
$password = $_POST['password'];
$website = $_POST['website'];
$user_data = [
'user_login' => $username,
'user_pass' => $password,
'user_url' => $website,
];
$user_id = wp_insert_user($user_data);
// success
if (!is_wp_error($user_id)) {
echo 'User created: ' . $user_id;
}
更新用户
wp_update_user(
mixed $userdata
);
更新数据库中的某个用户时,更新数据通过 $userdata 数据/对象中传递。如果需要更新用户元数据,使用 update_user_meta() 。
有关该函数参数的完整说明,请参阅 wp_update_user() 函数的参考文档。
更新用户示例
下面是更新用户网址字段的示例代码。
$user_id = 1;
$website = 'https://wordpress.org';
$user_id = wp_update_user(
[
'ID' => $user_id,
'user_url' => $website,
]
);
if (is_wp_error($user_id)) {
// error
} else {
// success
}
删除用户
wp_delete_user(
int $id,
int $reassign = null
);
删除用户时,我们可以选择把用户创建的内容重新分配给其他用户。
有关该函数参数的完整说明,请参阅 wp_delete_user() 函数的参考文档。
操作用户元数据
简介
WordPress 的 user 数据中仅包含用户的基本信息。
为了存储附加数据,WordPress 引入了 wp_usermeta 数据表,我们可以在这个数据表中存储任意多的用户数据。这个表使用用户 ID 字段和 wp_user 数据表关联。
操作用户元数据
在 WordPress 中,有两种方法可以操作用户元数据。
- 通过个人资料编辑界面中的表单
- 通过程序的方式使用相关函数操作
通过表单字段
使用表单字段更新用户元数据的方法适用于可以访问 WordPress 管理界面的情况,我们可以在用户资料编辑界面查看和编辑用户资料。
在我们深入研究一个示例之前,我们先了解一下这个过程中涉及的钩子以及为什么会有这些钩子非常重要。
edit_user_profile 钩子
只要用户编辑了自己的配置文件,就会触发此钩子,请记住,没有编辑自己个人资料的用户不会触发这个钩子。
show_user_profile 钩子
只要用户编辑了其他人的用户资料,就会触发此钩子。请记住,没有编辑其他人资料的用户不会触发此钩子。
示例表单
下面的例子中,我们将在用户资料编辑界面添加一个生日字段,并在用户资料更新时将其保存到数据库中。
<?php
/**
* The field on the editing screens.
*
* @param $user WP_User user object
*/
function wporg_usermeta_form_field_birthday($user)
{
?>
<h3>It's Your Birthday</h3>
<table class=form-table>
<tr>
<th>
<label for=birthday>Birthday</label>
</th>
<td>
<input type=date
class=regular-text ltr
id=birthday
name=birthday
value=<?= esc_attr(get_user_meta($user->ID, 'birthday', true)); ?>
title=Please use YYYY-MM-DD as the date format.
pattern=(19[0-9][0-9]|20[0-9][0-9])-(1[0-2]|0[1-9])-(3[01]|[21][0-9]|0[1-9])
required>
<p class=description>
Please enter your birthday date.
</p>
</td>
</tr>
</table>
<?php
}
/**
* The save action.
*
* @param $user_id int the ID of the current user.
*
* @return bool Meta ID if the key didn't exist, true on successful update, false on failure.
*/
function wporg_usermeta_form_field_birthday_update($user_id)
{
// check that the current user have the capability to edit the $user_id
if (!current_user_can('edit_user', $user_id)) {
return false;
}
// create/update user meta for the $user_id
return update_user_meta(
$user_id,
'birthday',
$_POST['birthday']
);
}
// add the field to user's own profile editing screen
add_action(
'edit_user_profile',
'wporg_usermeta_form_field_birthday'
);
// add the field to user profile editing screen
add_action(
'show_user_profile',
'wporg_usermeta_form_field_birthday'
);
// add the save action to user's own profile editing screen update
add_action(
'personal_options_update',
'wporg_usermeta_form_field_birthday_update'
);
// add the save action to user profile editing screen update
add_action(
'edit_user_profile_update',
'wporg_usermeta_form_field_birthday_update'
);
通过编程的方式
这种方法适合于我们构建自定义用户资料编辑界面,并打算禁止用户访问 WordPress 管理界面的情况。
可用于操作用户元数据的函数是:add_user_meta(), update_user_meta(), delete_user_meta() 和 get_user_meta()
添加
add_user_meta(
int $user_id,
string $meta_key,
mixed $meta_value,
bool $unique = false
);
有关该函数参数的完整说明,请参阅 add_user_meta() 的函数参考。
更新
update_user_meta(
int $user_id,
string $meta_key,
mixed $meta_value,
mixed $prev_value = ''
);
有关该函数参数的完整说明,请参阅 update_user_meta() 的函数参考。
删除
delete_user_meta(
int $user_id,
string $meta_key,
mixed $meta_value = ''
);
有关该函数参数的完整说明,请参阅 delete_user_meta() 的函数参考。
获取
get_user_meta(
int $user_id,
string $key = '',
bool $single = false
);
有关该函数参数的完整说明,请参阅 get_user_meta() 的函数参考。
注意,如果仅传递 $user_id 参数给该函数,该函数将获取该用户的所有元数据为一个关联数组。
我们可以在主题或插件的任何地方显示用户元数据。
角色和能力
角色和能力是 WordPress 中比较重要的两个概念,可以让我们控制用户权限。WordPress 将角色及其能力存储在 wp_options 数据据表中的 user_roles 字段中。
角色
角色为用户定义了一组能力,例如,用户可以在仪表盘中看到什么和操作什么。
默认情况下,WordPress 有 6 个角色。
- 超级管理员
- 管理员
- 编辑
- 作者
- 投稿者
- 订阅用户
我们可以添加自定义角色或删除默认角色。
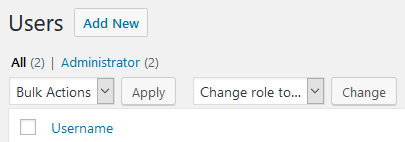
添加角色
我们可以使用 add_role() 添加新角色并为其分配能力。
function wporg_simple_role() {
add_role(
'simple_role',
'Simple Role',
[
'read' => true,
'edit_posts' => true,
'upload_files' => true,
]
);
}
// add the simple_role
add_action( 'init', 'wporg_simple_role' );
删除角色
我们可以使用 remove_role() 删除角色。
function wporg_simple_role_remove() {
remove_role( 'simple_role' );
}
// remove the simple_role
add_action( 'init', 'wporg_simple_role_remove' );
能力
能力定义了角色可以做什么,不可以做什么,如:编辑文章,发布文章等。
添加能力
我们可以为角色定义新能力。使用 get_role() 获取角色对象,然后使用 add_cap()
方法为角色添加新能力。
function wporg_simple_role_caps() {
// gets the simple_role role object
$role = get_role( 'simple_role' );
// add a new capability
$role->add_cap( 'edit_others_posts', true );
}
// add simple_role capabilities, priority must be after the initial role definition
add_action( 'init', 'wporg_simple_role_caps', 11 );
删除能力
我们可以从角色中移除某个能力。该实现类似于添加能力,不同之处在于使用的方法为 remove_cap() 。
使用角色和能力
获取角色
我们可以通过 get_role() 函数获取角色对象,包括它的所有能力。
get_role(
string $role
);
使用 user_can() 函数判断用户能力
我们可以使用 user_can() 函数检查用户是否有指定的角色或能力。
user_can(
int|object $user,
string $capability
);
使用 current_user_can() 判断当前登录用户的能力
current_user_can() 是 user_can() 的封装,使用当前用户对象作为 $user
参数。我们可以在前端或后端判断当前用户的能力。
示例
下面是一个在模板文件中添加编辑链接的实例,如果用户具有必要的能力,就显示文章编辑链接:
if (current_user_can('edit_posts')) {
edit_post_link('Edit', '<p>', '</p>');
}
多站点
在多站点中,使用 current_user_can_for_blog() 函数判断当前用户是否在某个站点上具有某个能力。
current_user_can_for_blog(
int $blog_id,
string $capability
);
参考
WordPress 用户角色和能力 参考文档。